Python: Numpy array data manipulation
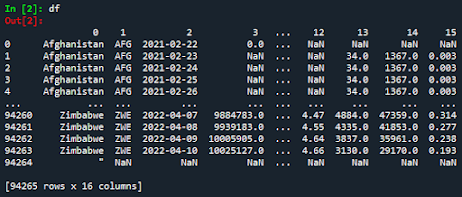
In the previous tutorial (please go to Python: Pandas DataFrame data manipulation ) , one method to manipulate a huge amount of data was explained. Now, in this tutorial, the second method of data manipulation will be explained. In this method, the library numpy is used which sorts data into arrays . But...what is an array ? An array is a data structure used to store data. To understand it better, let's remember what a matrix is. A matrix is a rectangular (sometimes square) array arranged in rows and columns. An example of a rectangular matrix can be the following: 1 2 3 4 5 6 7 8 As seen above, the matrix has 2 rows and 4 columns. In other words, it has dimensions 2x4. In case the number of rows and columns are the same, the matrix becomes square. In programming, if the matrix has only one row, it is commonly known as a row-vector, and if it has only one column, it is a column-vector. You can get one specific element of the matrix by knowing its position. ...